-
UID:2
-
- 注册时间2004-11-08
- 最后登录2025-07-04
- 在线时间7023小时
-
- 发帖11243
- 搜Ta的帖子
- 精华61
- 金钱150732
- 威望9968
- 贡献值702
- 好评度8190
-
访问TA的空间加好友用道具
|
从左向右自动 布局,自动换行,这个很有用。 头 文件FlowLayout.hpp - #ifndef FLOWLAYOUT_HPP
- #define FLOWLAYOUT_HPP
- #include <QtCore>
- #include <QtGui>
- class FlowLayout : public QLayout
- {
- public:
- FlowLayout(QWidget *parent, int margin = -1, int hSpacing = -1,
- int vSpacing = -1);
- FlowLayout(int margin = -1, int hSpacing = -1, int vSpacing = -1);
- ~FlowLayout();
-
- void addItem(QLayoutItem *item);
- int horizontalSpacing() const;
- int verticalSpacing() const;
- Qt::Orientations expandingDirections() const;
- bool hasHeightForWidth() const;
- int heightForWidth(int) const;
- int count() const;
- QLayoutItem *itemAt(int index) const;
- QSize minimumSize() const;
- void setGeometry(const QRect &rect);
- QSize sizeHint() const;
- QLayoutItem *takeAt(int index);
- private:
- int doLayout(const QRect &rect, bool testOnly) const;
- int smartSpacing(QStyle::PixelMetric pm) const;
-
- QList<QLayoutItem *> itemList;
- int m_hSpace;
- int m_vSpace;
- };
- #endif
CPP文件FlowLayout.cpp - #include <QtGui>
- #include "FlowLayout.hpp"
- FlowLayout::FlowLayout(QWidget *parent, int margin, int hSpacing,
- int vSpacing)
- : QLayout(parent), m_hSpace(hSpacing), m_vSpace(vSpacing)
- {
- setContentsMargins(margin, margin, margin, margin);
- }
- FlowLayout::FlowLayout(int margin, int hSpacing, int vSpacing)
- :m_hSpace(hSpacing), m_vSpace(vSpacing)
- {
- setContentsMargins(margin, margin, margin, margin);
- }
- FlowLayout::~FlowLayout()
- {
- QLayoutItem *item;
- while ((item = takeAt(0)))
- delete item;
- }
- void FlowLayout::addItem(QLayoutItem *item)
- {
- itemList.append(item);
- }
- int FlowLayout::horizontalSpacing() const
- {
- if (m_hSpace >= 0)
- {
- return m_hSpace;
- }
- else
- {
- return smartSpacing(QStyle::PM_LayoutHorizontalSpacing);
- }
- }
- int FlowLayout::verticalSpacing() const
- {
- if (m_vSpace >= 0)
- {
- return m_vSpace;
- }
- else
- {
- return smartSpacing(QStyle::PM_LayoutVerticalSpacing);
- }
- }
- int FlowLayout::count() const
- {
- return itemList.size();
- }
- QLayoutItem *FlowLayout::itemAt(int index) const
- {
- return itemList.value(index);
- }
- QLayoutItem *FlowLayout::takeAt(int index)
- {
- if (index >= 0 && index < itemList.size())
- return itemList.takeAt(index);
- else
- return 0;
- }
- Qt::Orientations FlowLayout::expandingDirections() const
- {
- return 0;
- }
- bool FlowLayout::hasHeightForWidth() const
- {
- return true;
- }
- int FlowLayout::heightForWidth(int width) const
- {
- int height = doLayout(QRect(0, 0, width, 0), true);
- return height;
- }
- void FlowLayout::setGeometry(const QRect &rect)
- {
- QLayout::setGeometry(rect);
- doLayout(rect, false);
- }
- QSize FlowLayout::sizeHint() const
- {
- return minimumSize();
- }
- QSize FlowLayout::minimumSize() const
- {
- QSize size;
- QLayoutItem *item;
- foreach (item, itemList)
- size = size.expandedTo(item->minimumSize());
- size += QSize(2*margin(), 2*margin());
- return size;
- }
- int FlowLayout::doLayout(const QRect &rect, bool testOnly) const
- {
- int left, top, right, bottom;
- getContentsMargins(&left, &top, &right, &bottom);
- QRect effectiveRect = rect.adjusted(+left, +top, -right, -bottom);
- int x = effectiveRect.x();
- int y = effectiveRect.y();
- int lineHeight = 0;
- QLayoutItem *item;
- foreach (item, itemList)
- {
- QWidget *wid = item->widget();
- int spaceX = horizontalSpacing();
- if (spaceX == -1)
- spaceX = wid->style()->layoutSpacing(
- QSizePolicy::PushButton, QSizePolicy::PushButton,
- Qt::Horizontal);
- int spaceY = verticalSpacing();
- if (spaceY == -1)
- spaceY = wid->style()->layoutSpacing(
- QSizePolicy::PushButton, QSizePolicy::PushButton,
- Qt::Vertical);
- int nextX = x + item->sizeHint().width() + spaceX;
- if (nextX - spaceX > effectiveRect.right() && lineHeight > 0)
- {
- x = effectiveRect.x();
- y = y + lineHeight + spaceY;
- nextX = x + item->sizeHint().width() + spaceX;
- lineHeight = 0;
- }
- if (!testOnly)
- item->setGeometry(QRect(QPoint(x, y), item->sizeHint()));
- x = nextX;
- lineHeight = qMax(lineHeight, item->sizeHint().height());
- }
- return y + lineHeight - rect.y() + bottom;
- }
- int FlowLayout::smartSpacing(QStyle::PixelMetric pm) const
- {
- QObject *parent = this->parent();
- if (!parent)
- {
- return -1;
- }
- else if (parent->isWidgetType())
- {
- QWidget *pw = static_cast<QWidget *>(parent);
- return pw->style()->pixelMetric(pm, 0, pw);
- }
- else
- {
- return static_cast<QLayout *>(parent)->spacing();
- }
- }
下面这个图是程序在960x600分辨率下自FlowLayout自适应每行 显示4个产品的截图 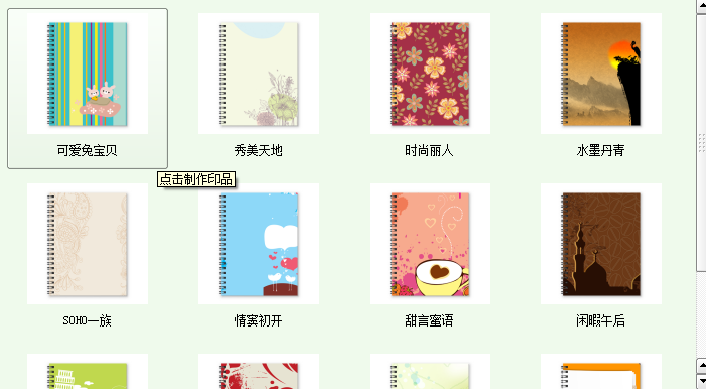 下面这个图是程序在1280x800分辨率下FlowLayout自适应每行显示5个产品的截图
|