前言按钮进度条,顾名思义,表面上长得像一个按钮,单击以后切换成进度条指示按钮单击动作执行的进度,主要用在一些需要直接在按钮执行动作
显示对应进度的场景,在很多网页中经常看到这种效果,这个效果有个优点就是直接在原地显示进度条,不占用其他位置,然后提供各种颜色可以设置。近期大屏电子看板程序接近尾声了,文章末尾贴出几张动图效果。
实现的功能 * 1:可设置进度线条宽度+颜色
* 2:可设置边框宽度+颜色
* 3:可设置圆角角度+背景颜色
效果图
头文件代码- #ifndef PROGRESSBUTTON_H
- #define PROGRESSBUTTON_H
- /**
- * 按钮进度条控件 作者:倪大侠(QQ:393320854 zyb920@hotmail.com) 2019-4-17
- * 1:可设置进度线条宽度+颜色
- * 2:可设置边框宽度+颜色
- * 3:可设置圆角角度+背景颜色
- */
- #include <QWidget>
- class QTimer;
- #ifdef quc
- #if (QT_VERSION < QT_VERSION_CHECK(5,7,0))
- #include <QtDesigner/QDesignerExportWidget>
- #else
- #include <QtUiPlugin/QDesignerExportWidget>
- #endif
- class QDESIGNER_WIDGET_EXPORT ProgressButton : public QWidget
- #else
- class ProgressButton : public QWidget
- #endif
- {
- Q_OBJECT
- Q_PROPERTY(int lineWidth READ getLineWidth WRITE setLineWidth)
- Q_PROPERTY(QColor lineColor READ getLineColor WRITE setLineColor)
- Q_PROPERTY(int borderWidth READ getBorderWidth WRITE setBorderWidth)
- Q_PROPERTY(QColor borderColor READ getBorderColor WRITE setBorderColor)
- Q_PROPERTY(int borderRadius READ getBorderRadius WRITE setBorderRadius)
- Q_PROPERTY(QColor bgColor READ getBgColor WRITE setBgColor)
- public:
- explicit ProgressButton(QWidget *parent = 0);
- protected:
- void resizeEvent(QResizeEvent *);
- void mousePressEvent(QMouseEvent *);
- void paintEvent(QPaintEvent *);
- void drawBg(QPainter *painter);
- void drawProgress(QPainter *painter);
- private:
- int lineWidth; //线条宽度
- QColor lineColor; //线条颜色
- int borderWidth; //边框宽度
- QColor borderColor; //边框颜色
- int borderRadius; //圆角角度
- QColor bgColor; //背景颜色
- double value; //当前值
- int status; //状态
- int tempWidth; //动态改变宽度
- QTimer *timer; //定时器改变进度
- public:
- int getLineWidth() const;
- QColor getLineColor() const;
- int getBorderWidth() const;
- QColor getBorderColor() const;
- int getBorderRadius() const;
- QColor getBgColor() const;
- QSize sizeHint() const;
- QSize minimumSizeHint() const;
- private slots:
- void progress();
- public Q_SLOTS:
- //设置线条宽度+颜色
- void setLineWidth(int lineWidth);
- void setLineColor(const QColor &lineColor);
- //设置边框宽度+颜色
- void setBorderWidth(int borderWidth);
- void setBorderColor(const QColor &borderColor);
- //设置圆角角度+背景颜色
- void setBorderRadius(int borderRadius);
- void setBgColor(const QColor &bgColor);
- Q_SIGNALS:
- void valueChanged(int value);
- };
- #endif // PROGRESSBUTTON_H
核心代码- void ProgressButton::paintEvent(QPaintEvent *)
- {
- QPainter painter(this);
- painter.setRenderHints(QPainter::Antialiasing | QPainter::TextAntialiasing);
- if (1 == status) {
- //绘制当前进度
- drawProgress(&painter);
- } else {
- //绘制按钮背景
- drawBg(&painter);
- }
- }
- void ProgressButton::drawBg(QPainter *painter)
- {
- painter->save();
- int width = this->width();
- int height = this->height();
- int side = qMin(width, height);
- QPen pen;
- pen.setWidth(borderWidth);
- pen.setColor(borderColor);
- painter->setPen(borderWidth > 0 ? pen : Qt::NoPen);
- painter->setBrush(bgColor);
- QRect rect(((width - tempWidth) / 2) + borderWidth, borderWidth, tempWidth - (borderWidth * 2), height - (borderWidth * 2));
- painter->drawRoundedRect(rect, borderRadius, borderRadius);
- QFont font;
- font.setPixelSize(side - 18);
- painter->setFont(font);
- painter->setPen(lineColor);
- painter->drawText(rect, Qt::AlignCenter, status == 2 ? "完 成" : "开 始");
- painter->restore();
- }
- void ProgressButton::drawProgress(QPainter *painter)
- {
- painter->save();
- int width = this->width();
- int height = this->height();
- int side = qMin(width, height);
- int radius = 99 - borderWidth;
- //绘制外圆
- QPen pen;
- pen.setWidth(borderWidth);
- pen.setColor(borderColor);
- painter->setPen(borderWidth > 0 ? pen : Qt::NoPen);
- painter->setBrush(bgColor);
- //平移坐标轴中心,等比例缩放
- QRect rectCircle(-radius, -radius, radius * 2, radius * 2);
- painter->translate(width / 2, height / 2);
- painter->scale(side / 200.0, side / 200.0);
- painter->drawEllipse(rectCircle);
- //绘制圆弧进度
- pen.setWidth(lineWidth);
- pen.setColor(lineColor);
- painter->setPen(pen);
- int offset = radius - lineWidth - 5;
- QRectF rectArc(-offset, -offset, offset * 2, offset * 2);
- int startAngle = offset * 16;
- int spanAngle = -value * 16;
- painter->drawArc(rectArc, startAngle, spanAngle);
- //绘制进度文字
- QFont font;
- font.setPixelSize(offset - 15);
- painter->setFont(font);
- QString strValue = QString("%1%").arg((int)value * 100 / 360);
- painter->drawText(rectCircle, Qt::AlignCenter, strValue);
- painter->restore();
- }
- void ProgressButton::progress()
- {
- if (0 == status) {
- tempWidth -= 5;
- if (tempWidth < this->height() / 2) {
- tempWidth = this->height() / 2;
- status = 1;
- }
- } else if (1 == status) {
- value += 1.0;
- if (value >= 360) {
- value = 360.0;
- status = 2;
- }
- } else if (2 == status) {
- tempWidth += 5;
- if (tempWidth > this->width()) {
- tempWidth = this->width();
- timer->stop();
- }
- }
- this->update();
- }
控件介绍 1. 超过145个精美控件,涵盖了各种仪表盘、进度条、进度球、指南针、曲线图、标尺、温度计、导航条、导航栏,flatui、高亮按钮、滑动选择器、农历等。远超qwt集成的控件数量。
2. 每个类都可以独立成一个单独的控件,零耦合,每个控件一个头文件和一个实现文件,不依赖其他文件,方便单个控件以源码形式集成到项目中,较少代码量。qwt的控件类环环相扣,高度耦合,想要使用其中一个控件,必须包含所有的代码。
3. 全部纯Qt编写,QWidget+QPainter绘制,支持Qt4.6到
Qt5.12的任何Qt版本,支持mingw、msvc、gcc等
编译器,不乱码,可直接集成到Qt Creator中,和自带的控件一样使用,大部分效果只要设置几个属性即可,极为方便。
4. 每个控件都有一个对应的单独的包含该控件源码的DEMO,方便参考使用。同时还提供一个所有控件使用的集成的DEMO。
5. 每个控件的源代码都有详细中文注释,都按照统一设计规范编写,方便学习自定义控件的编写。
6. 每个控件默认配色和demo对应的配色都非常精美。
7. 超过130个可见控件,6个不可见控件。
8. 部分控件提供多种样式风格选择,多种指示器样式选择。
9. 所有控件自适应窗体拉伸变化。
10. 集成自定义控件属性设计器,支持拖曳设计,所见即所得,支持导入导出xml格式。
11. 自带activex控件demo,所有控件可以直接运行在ie浏览器中。
12. 集成fontawesome图形字体+阿里巴巴iconfont收藏的几百个图形字体,享受图形字体带来的乐趣。
13. 所有控件最后生成一个dll动态库文件,可以直接集成到qtcreator中拖曳设计使用。
SDK下载 - SDK下载链接:
https://pan.baidu.com/s/1tD9v1YPfE2fgYoK6lqUr1Q 提取码:lyhk
- 自定义控件+属性设计器欣赏:
https://pan.baidu.com/s/1l6L3rKSiLu_uYi7lnL3ibQ 提取码:tmvl
- 下载链接中包含了各个版本的动态库文件,所有控件的头文件,使用demo。
- 自定义控件
插件开放动态库dll使用(永久免费),无任何后门和限制,请放心使用。
- 目前已提供26个版本的dll,其中包括了qt5.12.3 msvc2017 32+64 mingw 32+64 的。
- 不定期增加控件和完善控件,不定期更新SDK,欢迎各位提出建议,谢谢!
-
widget版本(QQ:517216493)
qml版本(QQ:373955953)三峰驼(QQ:278969898)。
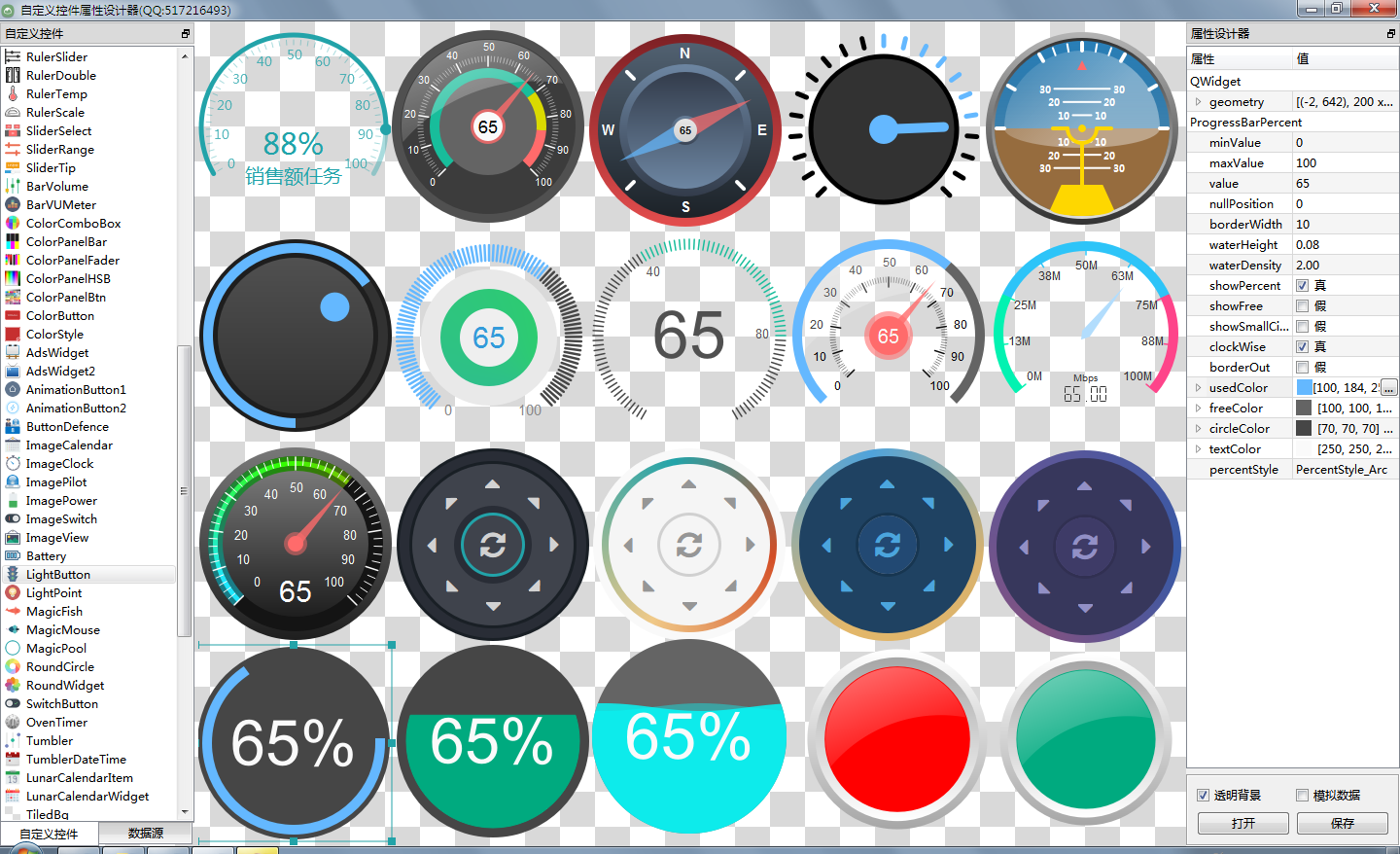