-
UID:110085
-
- 注册时间2010-12-21
- 最后登录2025-06-30
- 在线时间3568小时
-
- 发帖2843
- 搜Ta的帖子
- 精华2
- 金钱34104
- 威望3508
- 贡献值625
- 好评度3528
-
访问TA的空间加好友用道具
|
一个朋友需要定制一个 按钮, 部分需求如下: 1.可以设置圆角(Radius)值。 2.可以设置边框的粗细(BorderSize)和 颜色(BorderColor) 3.背景颜色有两种颜色RColor和PColor。 4.背景颜色 显示模式有3种: a)松开状态的颜色为RColor;按下状态的颜色为PColor。 b)松开状态的颜色为按键上半部分是RColor,按键下半部分为PColor;按下状态的颜色为按键上半部分是PColor,按键下半部分为RColor。 c)松开状态的颜色为RColor到PColor的渐变色;按下状态的颜色为按键上半部分是PColor到RColor的渐变色。 5.按键内部可以显示3行文字(Text[3]),并对每行文字设置 字体、对齐、大小、颜色。 6.可以处理鼠标按下、松开、移动、拖拽的 事件。 7.可以显示 图片,当显示图片时自动覆盖背景颜色和文字,但保留边框和圆角。 实现效果: 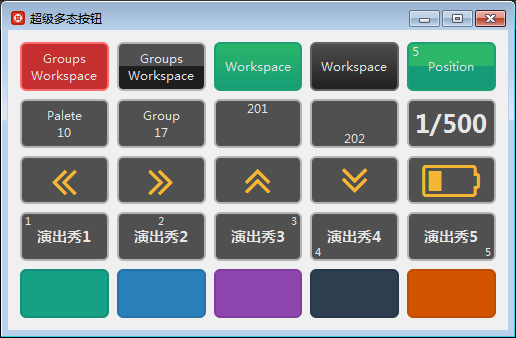 集成在QUC自定义控件中    核心代码: - void WKButton::paintEvent(QPaintEvent *)
- {
- //绘制准备工作,启用反锯齿
- QPainter painter(this);
- painter.setRenderHints(QPainter::Antialiasing | QPainter::TextAntialiasing);
- //绘制背景
- drawBg(&painter);
- //绘制文字
- drawText(&painter);
- }
- void WKButton::drawBg(QPainter *painter)
- {
- painter->save();
- //设置边框颜色及宽度
- QPen pen;
- pen.setColor(borderColor);
- pen.setWidthF(borderWidth);
- painter->setPen(pen);
- //绘制区域要减去边框宽度
- QRect rect;
- rect.setX(borderWidth);
- rect.setY(borderWidth);
- rect.setWidth(width() - borderWidth * 2);
- rect.setHeight(height() - borderWidth * 2);
- //如果背景图片存在则显示背景图片,否则显示背景色
- if (!bgImage.isNull()) {
- //等比例缩放绘制
- QPixmap img = bgImage.scaled(rect.width(), rect.height(), Qt::KeepAspectRatio, Qt::SmoothTransformation);
- painter->drawPixmap((this->rect().width() - img.width()) / 2, (this->rect().height() - img.height()) / 2, img);
- } else {
- if (colorMode == ColorMode_Normal) {
- if (isPressed) {
- painter->setBrush(QBrush(pressedColor));
- } else {
- painter->setBrush(QBrush(normalColor));
- }
- } else if (colorMode == ColorMode_Replace) {
- QLinearGradient gradient(QPoint(0, 0), QPoint(0, height()));
- if (isPressed) {
- gradient.setColorAt(0.0, pressedColor);
- gradient.setColorAt(0.49, pressedColor);
- gradient.setColorAt(0.50, normalColor);
- gradient.setColorAt(1.0, normalColor);
- } else {
- gradient.setColorAt(0.0, normalColor);
- gradient.setColorAt(0.49, normalColor);
- gradient.setColorAt(0.50, pressedColor);
- gradient.setColorAt(1.0, pressedColor);
- }
- painter->setBrush(gradient);
- } else if (colorMode == ColorMode_Shade) {
- QLinearGradient gradient(QPoint(0, 0), QPoint(0, height()));
- if (isPressed) {
- gradient.setColorAt(0.0, pressedColor);
- gradient.setColorAt(1.0, normalColor);
- } else {
- gradient.setColorAt(0.0, normalColor);
- gradient.setColorAt(1.0, pressedColor);
- }
- painter->setBrush(gradient);
- }
- painter->drawRoundedRect(rect, borderRadius, borderRadius);
- }
- painter->restore();
- }
- void WKButton::drawText(QPainter *painter)
- {
- if (!bgImage.isNull()) {
- return;
- }
- painter->save();
- //如果要显示角标,则重新计算显示文字的区域
- if (showSuperText) {
- int offset = 3;
- QRect rect;
- rect.setX(borderWidth * offset);
- rect.setY(borderWidth);
- rect.setWidth(width() - borderWidth * offset * 2);
- rect.setHeight(height() - borderWidth * 2);
- Qt::Alignment alignment = Qt::AlignCenter;
- if (superTextAlign == TextAlign_Top_Left) {
- alignment = Qt::AlignTop | Qt::AlignLeft;
- } else if (superTextAlign == TextAlign_Top_Center) {
- alignment = Qt::AlignTop | Qt::AlignHCenter;
- } else if (superTextAlign == TextAlign_Top_Right) {
- alignment = Qt::AlignTop | Qt::AlignRight;
- } else if (superTextAlign == TextAlign_Center_Left) {
- alignment = Qt::AlignLeft | Qt::AlignVCenter;
- } else if (superTextAlign == TextAlign_Center_Center) {
- alignment = Qt::AlignHCenter | Qt::AlignVCenter;
- } else if (superTextAlign == TextAlign_Center_Right) {
- alignment = Qt::AlignRight | Qt::AlignVCenter;
- } else if (superTextAlign == TextAlign_Bottom_Left) {
- alignment = Qt::AlignBottom | Qt::AlignLeft;
- } else if (superTextAlign == TextAlign_Bottom_Center) {
- alignment = Qt::AlignBottom | Qt::AlignHCenter;
- } else if (superTextAlign == TextAlign_Bottom_Right) {
- alignment = Qt::AlignBottom | Qt::AlignRight;
- }
- //绘制角标
- painter->setPen(superTextColor);
- painter->setFont(superTextFont);
- painter->drawText(rect, alignment, superText);
- }
- int offset = 5;
- QRect rect;
- rect.setX(borderWidth * offset);
- rect.setY(borderWidth);
- rect.setWidth(width() - borderWidth * offset * 2);
- rect.setHeight(height() - borderWidth * 2);
- Qt::Alignment alignment = Qt::AlignCenter;
- if (textAlign == TextAlign_Top_Left) {
- alignment = Qt::AlignTop | Qt::AlignLeft;
- } else if (textAlign == TextAlign_Top_Center) {
- alignment = Qt::AlignTop | Qt::AlignHCenter;
- } else if (textAlign == TextAlign_Top_Right) {
- alignment = Qt::AlignTop | Qt::AlignRight;
- } else if (textAlign == TextAlign_Center_Left) {
- alignment = Qt::AlignLeft | Qt::AlignVCenter;
- } else if (textAlign == TextAlign_Center_Center) {
- alignment = Qt::AlignHCenter | Qt::AlignVCenter;
- } else if (textAlign == TextAlign_Center_Right) {
- alignment = Qt::AlignRight | Qt::AlignVCenter;
- } else if (textAlign == TextAlign_Bottom_Left) {
- alignment = Qt::AlignBottom | Qt::AlignLeft;
- } else if (textAlign == TextAlign_Bottom_Center) {
- alignment = Qt::AlignBottom | Qt::AlignHCenter;
- } else if (textAlign == TextAlign_Bottom_Right) {
- alignment = Qt::AlignBottom | Qt::AlignRight;
- }
- painter->setPen(textColor);
- painter->setFont(textFont);
- painter->drawText(rect, alignment, text);
- painter->restore();
- }
|